The ADC

An analog-to-digital converter transforms a voltage on an analog input of the micro-controller into an integer number, which can be used in a program. The conversion follows a linear law.
Our micro-controller is equipped with a 10-bit converter, i.e. the integer result of the conversion will be between 0 et 210-1 =1023.
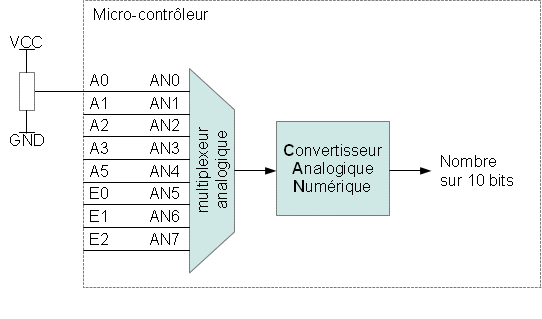
An analog-to-digital conversion takes some time; its result is not obtained immediately.
In order to operate an analog-to-digital conversion, so, it is necessary :
-
to position the analog multiplexer on the channel to convert
-
to wait for the necessary acquisition time for the voltage to stabilize
-
to start the conversion
-
to wait for the end of the conversion
-
to read the result of the conversion
All these operations take about 27µs.
A library of functions is provided to correctly configure the analog-to-digital converter and to execute conversions on the different channels without need to detail all the necessary steps.
In order to use the analog-to-digital converter library, we first need to put the following files in the project folder :
iut_adc.h
iut_adc.c
then we need to add them to the project.
After having included the header files of the library, it is necessary to initialize its utilization by a call to the function adc_init at the beginning of the program. The parameter to be passed to this initialization function corresponds to the number of the last analog channel used. We will pass “zero” in order to use only the AN0 channel, on which a potentiometer is connected.
Example:
#include <18f4550.h>
#include "iut_adc.h"
void main(void)
{
adc_init(0);
...
}
In order to operate a conversion, we need only to call the function adc_read passing as parameter the number of channel to convert (0 pour AN0, for example) and to store the result into a variable. A function call lasts for about 27µs.
Here is an example that operates the conversion of channel AN0, wired to the potentiometer, and displays the result on the ADC display, with two representations :
as an integer (from 0 to 1023) and in volt units (0 to 5 V)
#include <p18f4550.h>
#include "iut_ADC.h"
#include "iut_adc.h"
void main(void)
{
int potar = 0;
float v;
// initialisation
ADC_init();
adc_init(0);
ADC_position(0, 2);
ADC_putrs("IUT en ligne");
//infinite loop
while (1) {
potar = adc_read(0);
ADC_position(1, 0);
ADC_printf("%4d", potar);
v = potar * (5.0 / 1023);
ADC_position(1, 10);
ADC_printf("%5.3fV", v);
}
}
Note the use of the function ADC_printf to display :
-
For an int type variable of 4 characters (0 to 1023), we use the format %4d
-
For a float type variable, always positive, whose value is always positive and between 0 and 5 and with 3 decimal digits, we need at least 5 characters and we use the format %5.3f