To use the Timers

In order to count time or the occurrences of events, micro-controllers have specialized blocs called “timers”. A timer is basically a counter where we can adjust the clock, read and write the value. Timers are able to generate interruptions, but we will not address this topic in this class.
We will give an example of utilization of the Timer0 of our PIC18F4550. In order to program this timer, we will use the standard library provided by the C compiler. So, it is necessary to include the library header file timers.h, by using the following code:
#include<timers.h>
The function that allows to initialize the timers is: OpenTimer0. MPLAB C18 Libraries Documentation, Chapter 2.9
This function is documented in the MPLAB C18 Libraries Documentation, Chapter 2.9
Example of initialization of timer0:
OpenTimer0( TIMER_INT_OFF & // disable the interruptions
T0_16BIT & // 16-bit configuration of the timer
T0_SOURCE_INT & // use the internal clock
T0_PS_1_256 // divide the clock by 256
);
In our examples we will always disable interruptions. We will always use 16-bit configurated timers (counting range from 0 to 65535) since 8-bit timers provide a more limited counting range (0 to 255). We will use the internal clock for our electronic card and in this case the timer receives a clock having a frequency of 12 MHz. For the timer0 the possible values are 1, 2, 4, 8, 16, 32, 64, 128 ot 256.
When the timer is initialized, it counts continuously. It is necessary, hence, to be able to fix its value at a particular instant in the program, for example in order to set the value of the counter to zero. We will use the function WriteTimer0.
In order to set the timer to zero, one just needs to write:
WriteTimer0(0);
In order to read the timer,, the function ReadTimer0 returns its value. It is possible, in this way, to make a loop to wait for a certain elapsed time. By using the previous configuration, the timer counts at a frequency of 12 MHz divided by 256, that is 46875 Hz. Starting from zero, one second will have elapsed when the counter has reached 46875.
In order to wait for a second, we will write:
while (ReadTimer0() < 46875);
In the same way, in order to wait for half-a-second, it is necessary to reach 23438. We will write:
while (ReadTimer0() < 23438);
In order to have the led wired to A6 turn on/off intermittently at a frequency of 1 Hz (OFF during 0.5 s and ON 0.5 s), we can write the following program:
#include <p18f4550.h>
#include <timers.h>
void main(void)
{
// initialisation
TRISA &= 0xBF; // A6: output
OpenTimer0(
T0_PS_1_256 // divide the clock by 256
TIMER_INT_OFF & // disable the interruptions
T0_16BIT & // 16-bit configuration of the timer
T0_SOURCE_INT & // use the internal clock
);
// infinite loop
while (1) {
}WriteTimer0(0); // reset the timer to zero
while (ReadTimer0() < 23438); // wait for 0.5 s
PORTA |= 0x40; // turn ON the LED
WriteTimer0(0); // reset the timer to zero
while (ReadTimer0() < 23438); // wait for 0.5 s
PORTA &= 0xBF; // turn OFF the LED
}
Attention if the elapsed time does not seem to be realistic: the simulation might not be perfectly in real-time, it depends on the performances of your computer.
The elapsed time during the simulation from the point of view of the micro-controller is indicated in the bottom-left corner of the simulator window.
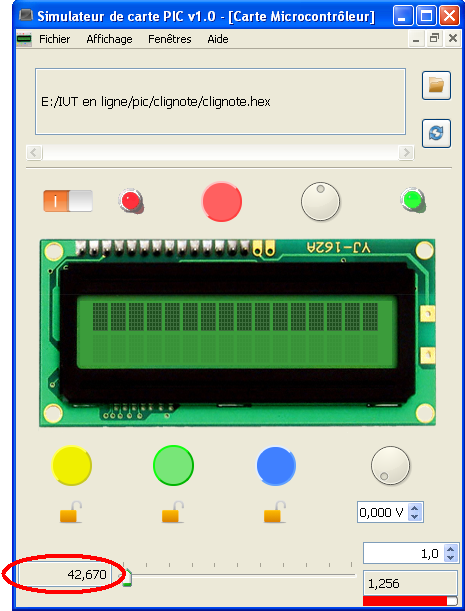