Libraries

- Bitwise AND : &
- Bitwise OR : | (Alt Gr + 6 on an AZERTY keyboard)
- Bitwise complementation : ~
- Comparison “equal to” : ==
- Comparison “not equal to” : !=

Let’s take a variable a whose size is one byte (8 bits, which are 8 different physical electrical wires).
Definition of a :
The variable a is composed by 8 bits. The bit 7 is the most significant bit (MSB), while the bit 0 is the less significant bit (LSB).
For example, if a is 0x75 in hexadecimal notation, which is 117 in decimal notation, we have :
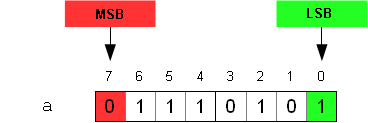

In order to test a single bit, we operate an AND operation and we compare the result to zero.
We build the mask by putting to 1 the bit to be tested.
If we want to test if the bit value is 1 we will operate a comparison « no equal to » zéro.
If we want to test if the bit value is zero, we will operate a comparison « equal to » zéro.
Examples :
- If we want to test that the value of the bit 3 is equal to 1 we write :
if ( (a & 0x08) != 0) {
// instructions to execute if the value of the bit 3 is 1
}
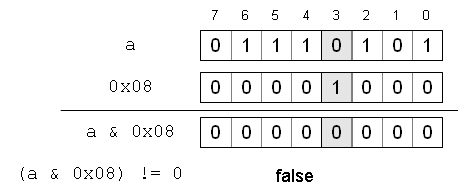
Here, the test result is false, since the value of the bit 3 is 0.
- If we had tested whether the bit 4 was equal to 1, we would have obtained:
if ( (a & 0x10) != 0) {
// Instructions to execute if the value of the bit 4 is 1
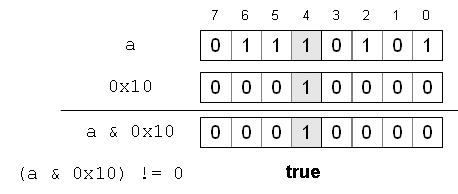
Here, the test result is true, since the value of the bit 4 is 1.
- In order to test if the value of the bit 1 is 0, we write :
if ( (a & 0x02) == 0) {
// Instructions to execute if the value of the bit 1 is 0
}
Here the test result is true, since the value of the bit 1 is 0.
- In order to test if the value of the bit 6 is 0, we write :
if ( (a & 0x40) == 0) {
// Instructions to execute if the value of the bit 6 is 0
}
Here, the test result is false, since the value of the bit 6 is 1.
Here, we have learnt how to test a single bit. It is possible to test at once multiple bits of a byte.